UI
ScrollView
<ScrollView>
is a UI component that shows a scrollable content area. Content can be scrolled vertically or horizontally.
It's important to note that <ScrollView>
extends ContentView
, so it can only have a single child element.
By default, ScrollView scrolls vertically. To scroll horizontally, set the ScrollView's orientation
property to horizontal
.
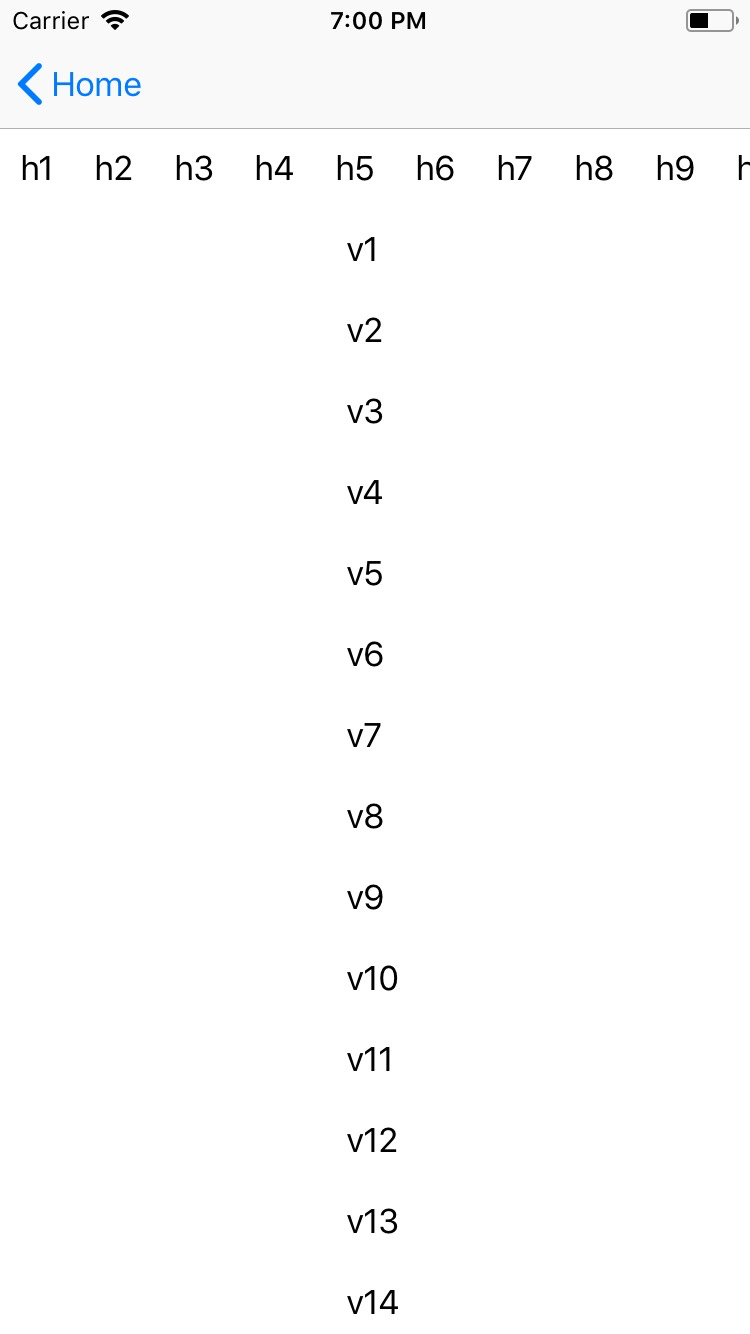
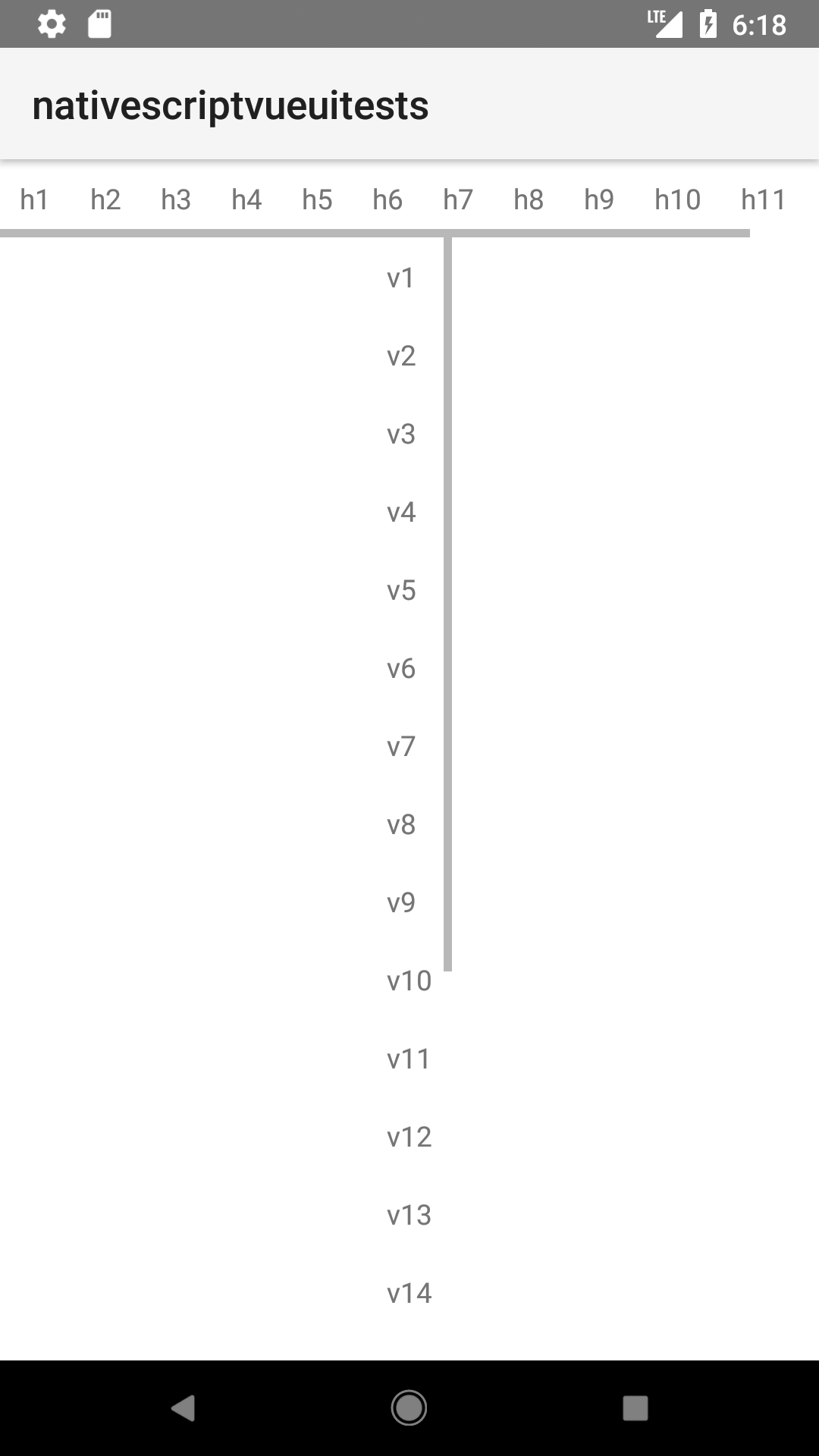
Creating a Simple ScrollView
<ScrollView scroll="onScroll">
<GridLayout rows="200 200 200 200 200 200 200 200 200 200">
<Label row="0" text="Some row content goes here..." />
<Label row="1" text="Some row content goes here..." />
<Label row="2" text="Some row content goes here..." />
<Label row="3" text="Some row content goes here..." />
<Label row="4" text="Some row content goes here..." />
<Label row="5" text="Some row content goes here..." />
<Label row="6" text="Some row content goes here..." />
<Label row="7" text="Some row content goes here..." />
<Label row="8" text="Some row content goes here..." />
<Label row="9" text="Some row content goes here..." />
</GridLayout>
</ScrollView>
import { Page, ScrollEventData, ScrollView } from '@nativescript/core'
export function onScroll(args: ScrollEventData) {
const scrollView = args.object as ScrollView
console.log('scrollX: ' + args.scrollX)
console.log('scrollY: ' + args.scrollY)
}
Props
orientation
<ScrollView orientation="horizontal">
Gets or sets the direction in which the content can be scrolled: horizontal
or vertical
. Defaults to vertical
.
scrollBarIndicatorVisible
<ScrollView scrollBarIndicatorVisible="false">
Specifies if the scrollbar is visible. Defaults to true
.
isScrollEnabled
<ScrollView isScrollEnabled="false">
Gets or sets a value indicating whether scroll is enabled.
verticalOffset
verticalOffset: number = scrollView.verticalOffset
Gets a value that contains the vertical offset of the scrolled content.
horizontalOffset
horizontalOffset: number = scrollView.horizontalOffset
Gets a value that contains the horizontal offset of the scrolled content.
scrollableHeight
scrollableHeight: number = scrollView.scrollableHeight
Gets the maximum value for the verticalOffset.
scrollableWidth
scrollableWidth: number = scrollView.scrollableWidth
Gets the maximum value for the horizontalOffset.
scrollToVerticalOffset()
scrollView.scrollToVerticalOffset(value: number, animated: boolean)
Scrolls the content to the specified vertical offset position. Set animated
to true
for animated scroll, false
for immediate scroll.
scrollToHorizontalOffset()
scrollView.scrollToHorizontalOffset(value: number, animated: boolean)
Scrolls the content to the specified horizontal offset position. Set animated
to true
for animated scroll, false
for immediate scroll.
Event(s)
scroll
Emitted when a scroll event occurs. For the event's data, see ScrollEventData Interface.
Native component
Android | iOS |
---|---|
android.view | UIScrollView |