UI
TabView
<TabView>
is a navigation component that shows content grouped into tabs and lets users switch between tabs.
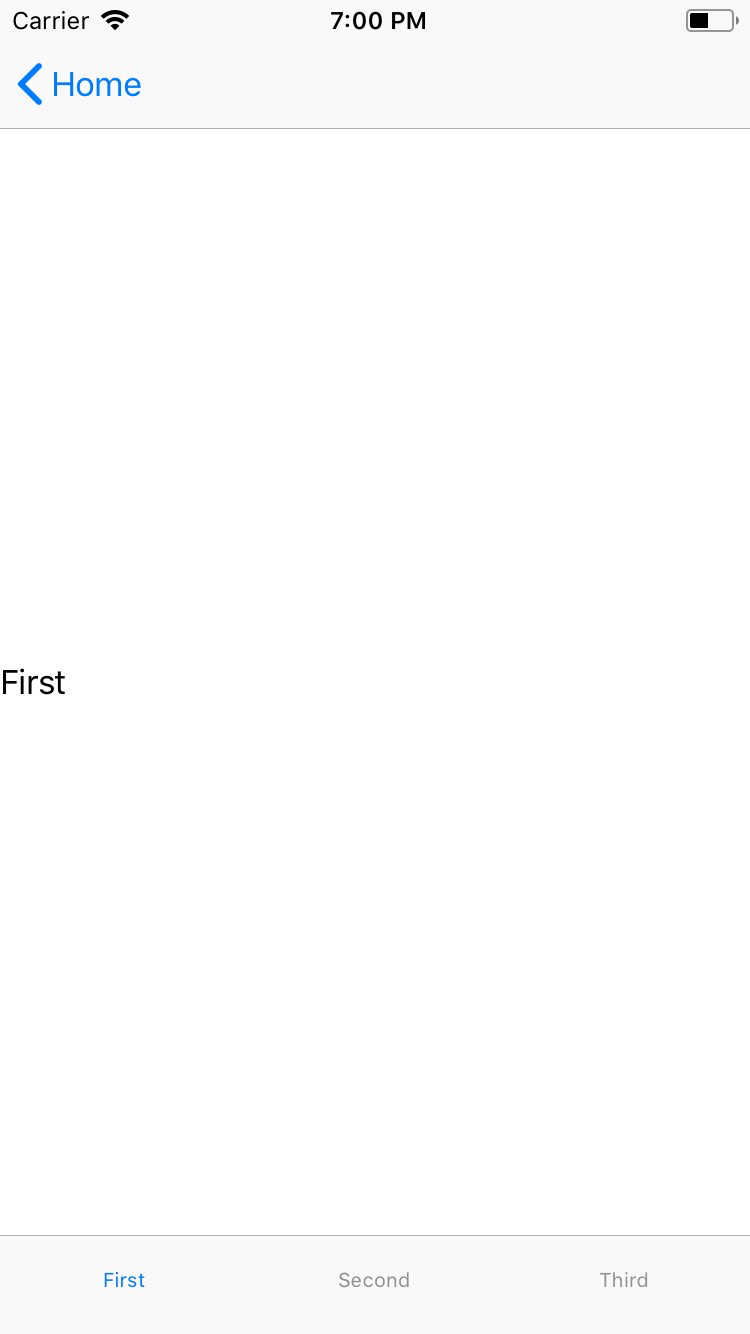
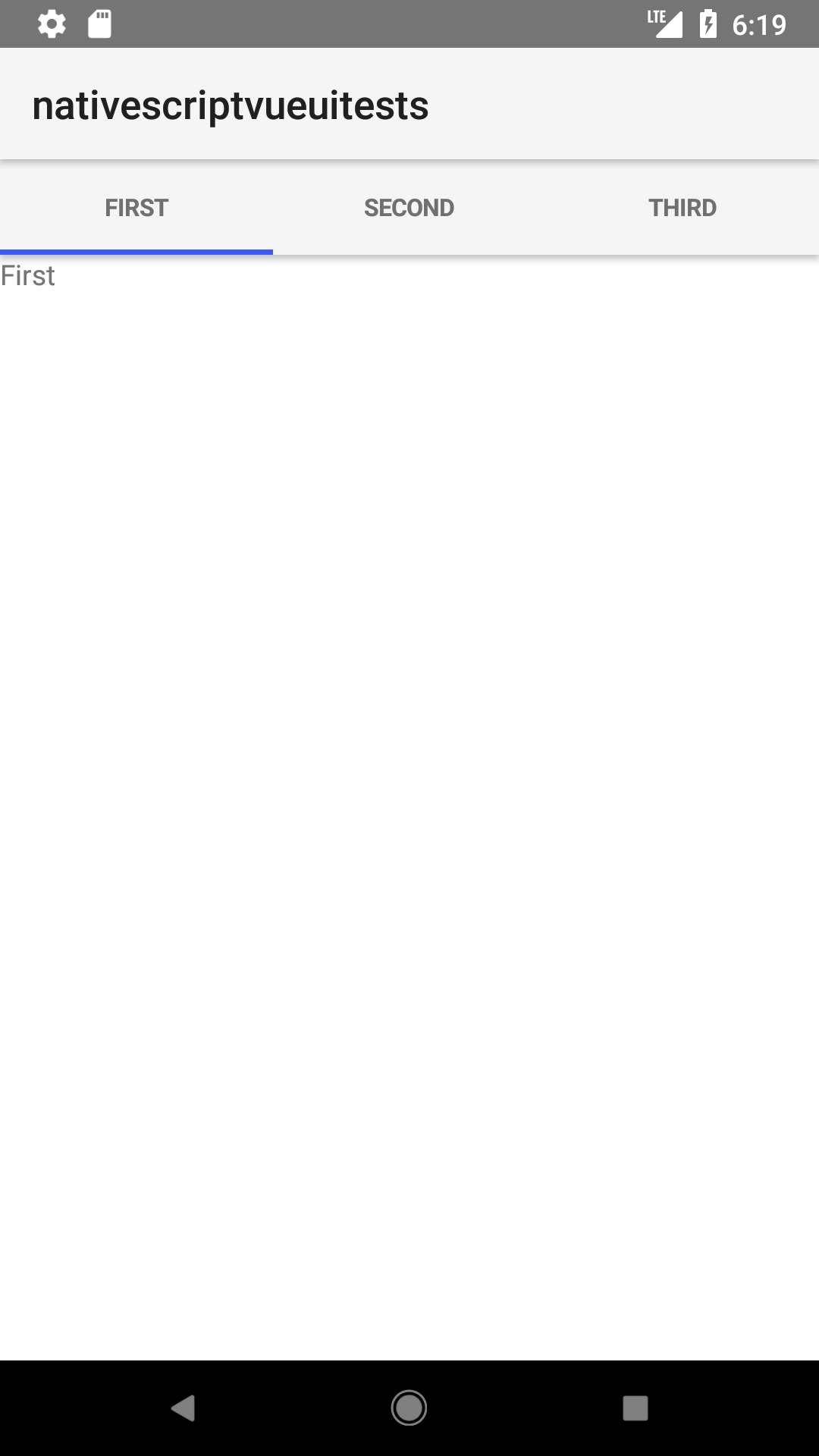
Simple TabView
<TabView
selectedIndex="{{ tabSelectedIndex }}"
selectedIndexChanged="{{ onSelectedIndexChanged }}"
androidTabsPosition="bottom"
androidOffscreenTabLimit="0"
tabBackgroundColor="#3d5a80"
tabTextColor="#fff"
selectedTabTextColor="#eae2b7">
<TabViewItem title="Profile">
<StackLayout>
<Label text="Change Tab" class="text-center text-2xl font-bold pt-4" />
<Label text="{{ tabSelectedIndexResult }}" class="mt-4 text-center" textWrap="true" />
</StackLayout>
</TabViewItem>
<TabViewItem title="Stats">
<StackLayout>
<Label text="Change Tab" class="text-center text-2xl font-bold pt-4" />
<Label text="{{ tabSelectedIndexResult }}" class="mt-4 text-center" textWrap="true" />
</StackLayout>
</TabViewItem>
<TabViewItem title="Settings">
<StackLayout>
<Label text="Change Tab" class="text-center text-2xl font-bold pt-4" />
<Label text="{{ tabSelectedIndexResult }}" class="mt-4 text-center" textWrap="true" />
</StackLayout>
</TabViewItem>
</TabView>
import {
Observable,
SelectedIndexChangedEventData
} from '@nativescript/core'
export class HelloWorldModel extends Observable {
tabSelectedIndex = 0
tabSelectedIndexResult = 'Profile Tab (tabSelectedIndex = 0 )'
....
onSelectedIndexChanged(args: SelectedIndexChangedEventData){
if (args.oldIndex !== -1) {
const newIndex = args.newIndex
if (newIndex === 0) {
this.set('tabSelectedIndexResult', 'Profile Tab (tabSelectedIndex = 0 )')
} else if (newIndex === 1) {
this.set('tabSelectedIndexResult', 'Stats Tab (tabSelectedIndex = 1 )')
} else if (newIndex === 2) {
this.set('tabSelectedIndexResult', 'Settings Tab (tabSelectedIndex = 2 )')
}
}
}
}
Tip
You can use images for tab icons instead of icon fonts. For more information about how to control the size of icons, see Working with image from resource folders.
Styling
The TabView
component has the following unique styling properties:
Props
selectedIndex
<TabView selectedIndex="{{ tabSelectedIndex }}" />
selectedIndex = tabView.selectedIndex
Gets or sets the currently selected tab. Default is 0
.
tabTextColor
<TabView tabTextColor="#fff" />
Corresponding CSS: tab-text-color
.tab-view {
tab-text-color: #fff;
}
Gets or sets the text color of the tabs titles.
tabTextFontSize
Corresponding CSS: tab-text-font-size
.tab-view {
tab-text-font-size: 24;
}
Gets or sets the font size of the tabs titles.
tabBackgroundColor
<TabView tabBackgroundColor="#3d5a80">
Corresponding CSS: tab-background-color
.tab-view {
tab-background-color: #3d5a80;
}
Sets the background color of the tabs.
androidSelectedTabHighlightColor
<TabView androidSelectedTabHighlightColor="#76ABEB">
Corresponding CSS: android-selected-tab-highlight-color
.tab-view {
android-selected-tab-highlight-color: #76abeb;
}
(Android-only
)Sets the underline color of the tabs.
androidTabsPosition
<TabView androidTabsPosition="top" >
Sets the position of the TabView in Android platform.
Valid values: top
or bottom
. Defaults to top
.
iosIconRenderingMode
Gets or sets the icon rendering mode(UIImage.RenderingMode) on iOS.
Defaults to automatic
.
Valid values are: automatic
| alwaysOriginal
| alwaysTemplate
...Inherited
For additional inherited properties, refer to the API Reference.
TabViewItem Props
title
<TabViewItem title="Profile">
Gets or sets the title of the tab strip entry.
textTransform
<TabViewItem title="Profile" textTransform="capitalize" />
Corresponding CSS: text-transform
.tab-view-item {
text-transform: capitalize;
}
Sets the text transform individually for every TabViewItem
. See textTransformType for valid options.
iconSource
<TabViewItem title="Profile"
iconSource="~/assets/images/cat.jpeg" >
Gets or sets the icon source of the tab strip entry. Supports local image paths (~
), resource images (res://
) and icon fonts (font://
).
Event(s)
selectedIndexChange
<TabView loaded="{{ onTabViewLoaded }}" >
onTabViewLoaded(args: EventData){
const tabView = args.object as TabView
tabView.on("selectedIndexChanged",(args: SelectedIndexChangedEventData)=>{
})
}
Raised when the selected index changes.
SelectedIndexChangedEventData
The SelectedIndexChangedEventData
object provides the following data:
Name | Type | Description |
---|---|---|
oldIndex | number | The old selected index. |
newIndex | number | The new selected index. |
Native component
Android | iOS |
---|---|
android.support.v4.view.ViewPager | UITabBarController |