Note: You are on the beta version of our docs. This is a work in progress and may contain broken links and pages.
UI
SearchBar
<SearchBar>
is a UI component that provides a user interface for entering search queries and submitting requests to the search provider.
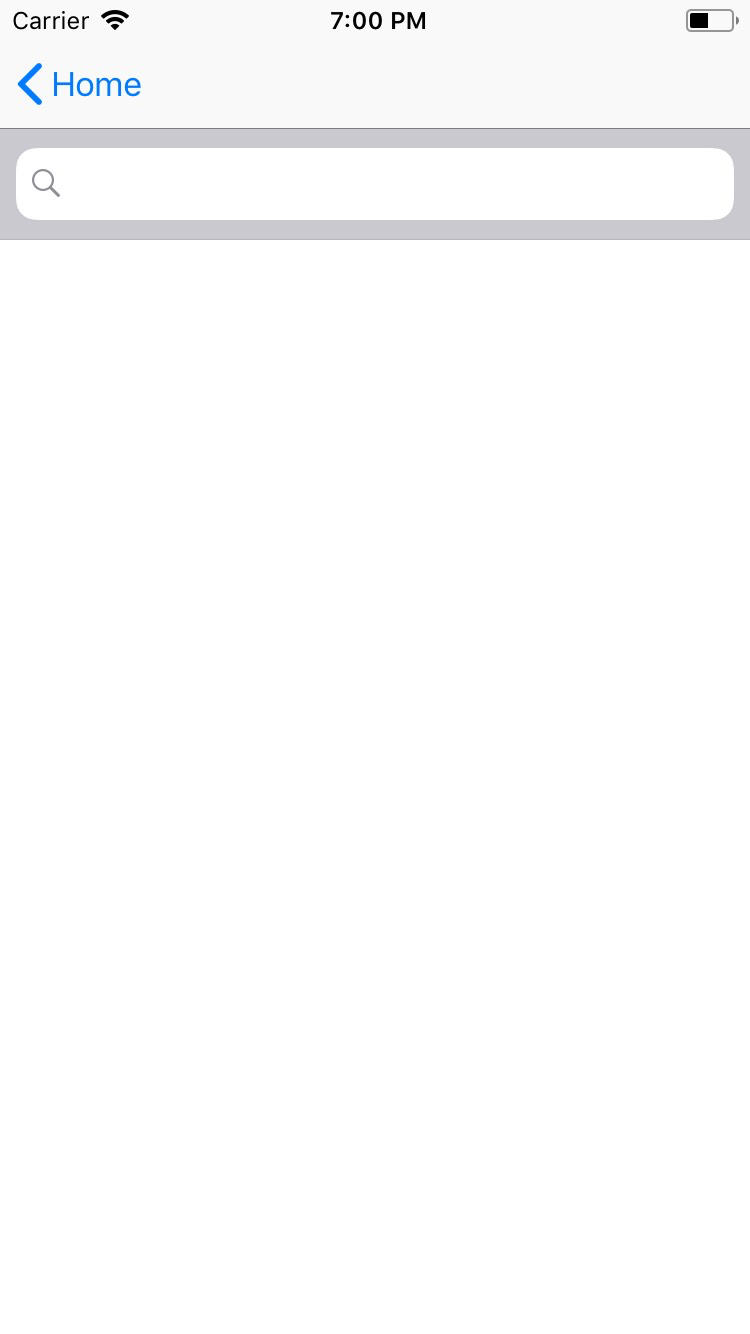
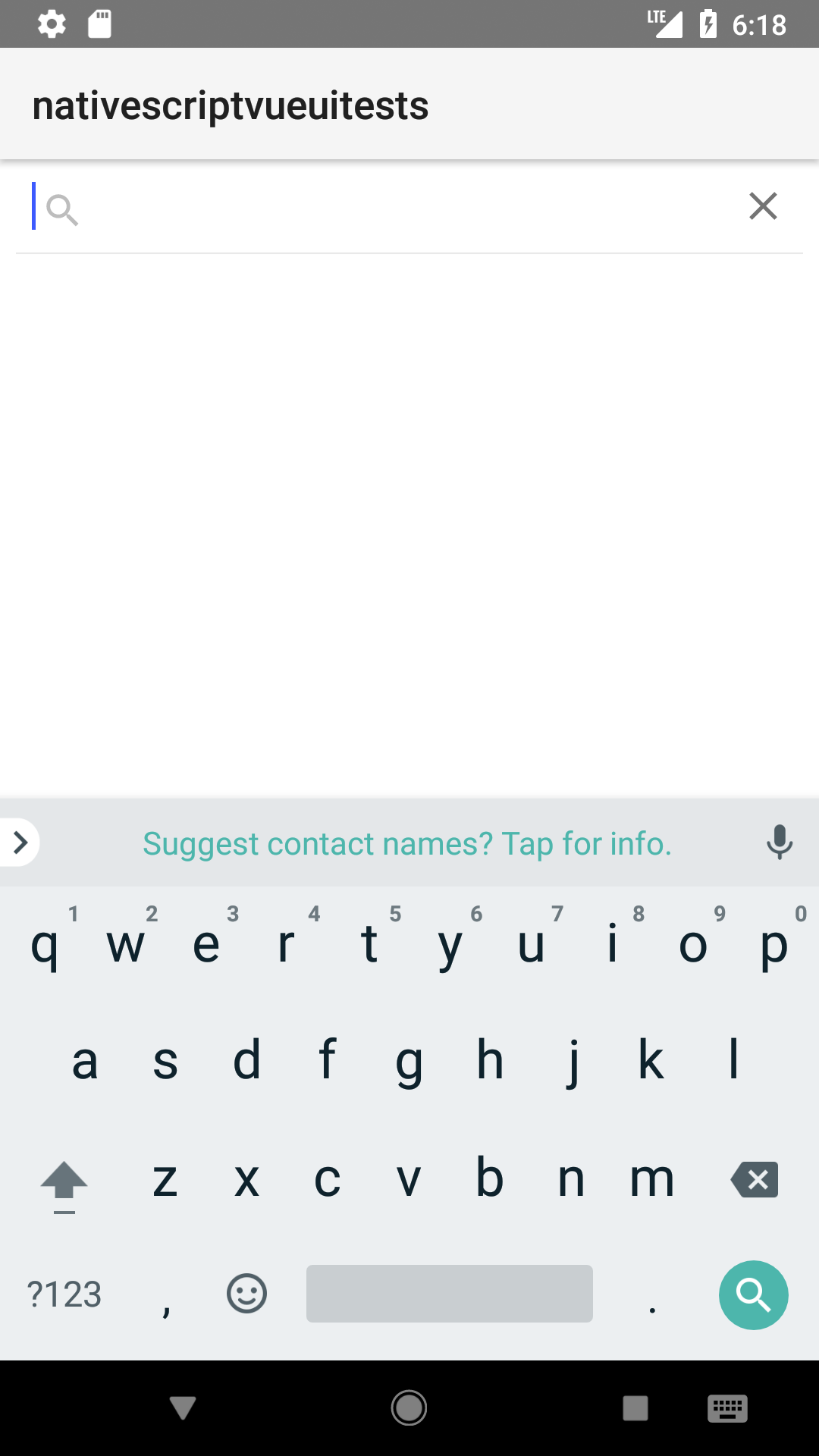
A simple SearchBar handling the clear and submit events
xml
<SearchBar id="searchBar"
hint="Enter search term here ..."
text="{{ searchText }}" clear="{{ onClear }}"
submit="{{ onSubmit }}"
loaded="{{ onSearchBarLoaded }}"/>
ts
import { Observable, Page, SearchBar } from '@nativescript/core'
export function onNavigatingTo(args) {
const page = args.object as Page
const vm = new HelloWorldModel()
page.bindingContext = vm
}
export class HelloWorldModel extends Observable {
searchText = ''
constructor() {
super()
}
onSearchBarLoaded(args: EventData) {
const searchBar = args.object as SearchBar
searchBar.on('textChange', (args: PropertyChangeData) => {
console.log('Event name: ', args.eventName)
})
}
onSubmit(args: EventData) {
const searchBar = args.object as SearchBar
console.log(`Searching for ${searchBar.text}`)
}
onClear(args: EventData) {
const searchBar = args.object as SearchBar
console.log(`Clear event raised`)
}
}
Props
hint
xml
<SearchBar hint="Enter search term here ..." />
Gets or sets placeholder text for the input area.
text
xml
<SearchBar text="{{ searchText }}" />
Gets or sets the value of the search query.
textFieldBackgroundColor
xml
<SearchBar textFieldBackgroundColor="#76ABEB"/>
Gets or sets the background color of the input area.
textFieldHintColor
xml
<SearchBar textFieldHintColor="#fff"/>
Gets or sets the color of the placeholder text.
...Inherited
For additional inherited properties, refer to the API Reference
Event(s)
textChange
ts
searchBar.on('textChange', (args: PropertyChangeData) => {
console.log('Event name: ', args.oldValue)
})
Emitted when the search text is changed.
submit
xml
<SearchBar submit="{{ onSubmit }}" />
ts
export class HelloWorldModel extends Observable {
onSubmit(args: EventData) {
const searchBar = args.object as SearchBar
console.log(`Searching for ${searchBar.text}`)
}
}
Emitted when the search text is submitted.
clear
xml
<SearchBar clear="{{ onClear }}" />
ts
export class HelloWorldModel extends Observable {
onClear(args: EventData) {
const searchBar = args.object as SearchBar
console.log(`Clear event raised`)
}
}
Emitted when the current search input is cleared through the X button in the input area.
Native Component
Android | iOS |
---|---|
android.widget.SearchView | UISearchBar |
- Previous
- ScrollView
- Next
- SegmentedBar